Learning Owl Framework - Component Props
Component props are the properties that are passed to a component. They are used to pass data to a component.
What you will learn
- How to pass props to a component
- How to read props from a component
- How to specify default values for props
- How props are changed
- What happens when props are changed
Owl components use props to represent the properties of a component and more importantly to communicate with other sub-components. Every parent component can provide some information to its child components by specifying props parameters similar to HTML attributes, but in props, you can pass objects and arrays or even functions.
Let’s observe the following example in which we are going to create an Avatar of an image that represents the profile picture of someone.
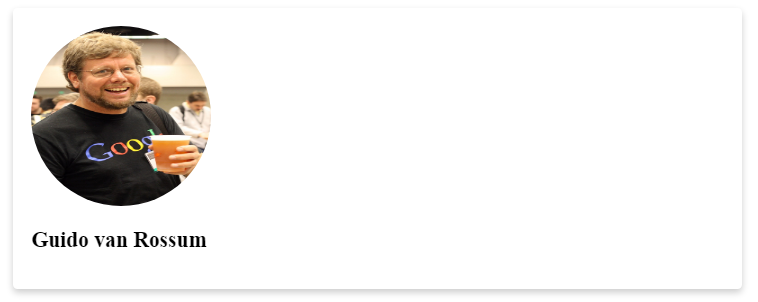
import { Avatar } from './Profile';
import { Component, xml } from '@odoo/owl';
export class Root extends Component {
static template = xml`
<div id="app">
<div class="card">
<Avatar src="'https://upload.wikimedia.org/wikipedia/commons/thumb/6/66/Guido_van_Rossum_OSCON_2006.jpg/800px-Guido_van_Rossum_OSCON_2006.jpg'"
width="600"
height="400"
name="'Guido van Rossum'"/>
<h2>Guido van Rossum</h2>
</div>
</div>
`;
static components = { Avatar };
}
We used <Avatar>
Component and we did pass to it some props attributes, for example,
- src image URL property.
- width width property of an image.
- height height property of an image.
- name name of the person.
So in detail how the Avatar component is actually written:
import { Component, xml } from '@odoo/owl';
export class Avatar extends Component {
static template = xml`
<img
class="circle-avatar-200"
t-att-src="props.src"
t-att-width="props.width"
t-att-height="props.height"
t-att-alt="props.name"/>
`;
setup() {
console.log(this.props);
}
}
📑Note What we did is simply pass props from Root component to a child component and log these props inside Avatarcomponent.
{
src:"https://upload.wikimedia.org/wikipedia/commons/thumb/6/66/Guido_van_Rossum_OSCON_2006.jpg/800px-Guido_van_Rossum_OSCON_2…",
width: 600,
height: 400,
name: "Guido van Rossum"
}
Specifying a default value for a prop
A default value can be passed for props so it can fall back to use this value if it is not specified.
import { Component, xml } from '@odoo/owl';
export class Avatar extends Component {
/** Component **/
}
Avatar.defaultProps = {
src: 'https://storage.googleapis.com/proudcity/mebanenc/uploads/2021/03/placeholder-image.png',
width: '300',
height: '300',
};
The previous example shows that whenever the image profile is not specified, it will hold the default value from defaultProps static attribute in this case the profile will look something like this:
What happens when props are changed
Props are attributes that represent a component-specific property that is designed according to what the component may be used for and it can be passed from the outside world when the component is mounted to the DOM.
❓So what happens when the props of a component are changed?
Whenever a props change takes place, a re-render is triggered by the component hooks by calling the render method internally by owl so it will update the dom with the new values for better compositionally there is no need to update the dom.
Plug and play online
Recap
- Props can be passed as HTML attributes to components.
- You can specify default props for a class component.
- owl uses
props
anddefaultProps
as a static class attribute. - this.props is totally different from
Component.props
. - props are not changed unless we add state management to them.
- props of a component can be interactive with state management.